It is quite a powerful API for text to speech, however it is not very well documented as it is in a private framework. To test out the API follow the following steps:
1. Create the VSSpeechSynthesizer.h file and add it into the project.
Code:#import <foundation/foundation.h> @interface VSSpeechSynthesizer : NSObject { } + (id)availableLanguageCodes; + (BOOL)isSystemSpeaking; - (id)startSpeakingString:(id)string; - (id)startSpeakingString:(id)string toURL:(id)url; - (id)startSpeakingString:(id)string toURL:(id)url withLanguageCode:(id)code; - (float)rate; // default rate: 1 - (id)setRate:(float)rate; - (float)pitch; // default pitch: 0.5 - (id)setPitch:(float)pitch; - (float)volume; // default volume: 0.8 - (id)setVolume:(float)volume; @end |
2. Create and initiate the VSSpeechSynthesizer object in the appropiate area of the code.
Code:VSSpeechSynthesizer *speech = [[NSClassFromString(@"VSSpeechSynthesizer") alloc] init];
3. Adjust the pitch, rate or volume accordingly.
Eg:[speech setRate:(float)1.0];
4. Create the speech in the appropiate area of the code.
Eg:[speech startSpeakingString:@"Hello world, how are you"];
5. Include the private VoiceServices into the frameworks
Eg: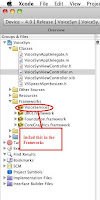
Depending on where you install the IPhone SDK, the location of the VoiceServices framework may varies. Usually the VoiceServices framework is in the following directory:
/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS4.0.sdk
/System/Library/PrivateFrameworks/VoiceServices.framework/VoiceServices
*Note: The VoiceServies framework only available in the iPhoneOS platform, hence it can only build and run on the actual iPhone device. It can not be run and test on the simulator.
Sample code:
I've downloaded your sample project and installed it on my iPhone 4. The app loads fine, but it doesn't speak.
ReplyDeleteAm I missing something?
Just for the record, I'm an idiot, it works perfect. Volume was down.
ReplyDeleteThank you so much. My son doesn't speak and I'm not a fan of the available "talker" apps for children. So I'm writing my own app for him. I used your sample code and it works great. You don't happen to know if there is any way to change the voice to sound like a male speaker instead?
ReplyDeleteHi, I tried to build this into a sample app, but can't seem to get it to speak. Can you repost your Sample Project.zip, as it's no longer available. Thanks.
ReplyDeleteIs it works on iPhone 5 or its updated version? ucuz iphone 4
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteNice and very helpful information i have got from your post. Even your whole blog is full of interesting information which is the great sign of a great blogger.
ReplyDeleteLenovo - IdeaPad 15.6" Laptop - 6GB Memory - 1TB Hard Drive - Dark Chocolate
Lenovo - 14" Notebook - 4 GB Memory - 320 GB Hard Drive - Black